2017年12月31日
《その214》 クロスキャスト(2) & p.259演習7-3
クロスキャスト
クラス C は、多重継承により、クラス A から純粋仮想関数 fa を、クラス B から純粋仮想関数 fb を受け継いでいます。下のプログラムです。
クラス C のオブジェクトは、2つの整数値 x, y をデータメンバに持ち、
関数 fa は和 x+y を、
関数 fb は差 x - y を、
それぞれ返却します。
main関数では、C型オブジェクトを3つ生成して、それらを、
A*型のポインタ a[0], a[1], a[2] が指しています。
その結果、例えば、
A[0]->fa();
とすれば、メンバ関数 fa を呼び出すことができます。しかし、
A[0]->fb();
でメンバ関数 fb を呼び出すことはできません。
関数 fb は、クラス A からではなく、クラス B から受け継いだものだからです。
そこで、ポインタ A[0] を A*型から B*型にキャストすることにします。
クラス A とクラス B は、まったく別のクラスで、親子関係もありませんから、本来であればキャストは不可能です。
ところが、 クラス A, B には、共通の子クラス C が存在します。
このようなときには、dynamic_cast<>演算子を用いてキャストを成功させることができます。
この形式のキャストは、 クロスキャストと呼ばれます 。
他人だったときにはキャストできなかった男女 A, B に、共通の子供 C ができると、A, B は、もう他人ではないのでキャストが可能になる、といった感じでしょうか (∩。∩;)ゞ
B* p = dynamic_cast<B*>(a[0]);
p->fb();
で、メンバ関数 fb を呼び出すことが可能になります。
// ------------------------------------
#include <iostream>
using namespace std;
class A {
public:
virtual int fa() = 0;
};
class B {
public:
virtual int fb() = 0;
};
class C : public A, public B {
int x;
int y;
public:
C(int s, int t) : x(s), y(t) { }
int fa() { return x + y; }
int fb() { return x - y; }
};
int main()
{
A* a[] = {
new C(20, 10),
new C(50, 30),
new C(66, 33),
};
for (int i = 0; i < 3; i++)
cout << a[i]->fa() << " ";
cout << '\n';
for (int i = 0; i < 3; i++) {
B* p = dynamic_cast<B* >(a[i]);
cout << p->fb() << " ";
}
cout << '\n';
for (int i = 0; i < 3; i++)
delete a[i];
}
// ------------------------------------

新版明解C++中級編 p.259 演習7-3
次のプログラムに、左上直角の二等辺三角形 RectEquilTriangleLU と右下直角の二等辺三角形 RectEquilTriangleRB を作成して加えよ。
// ------------------------------------
#include <string>
#include <sstream>
#include <iostream>
// 図形クラス(抽象クラス)
class Shape {
public:
virtual ~Shape() = 0;
virtual Shape* clone() const = 0;
virtual void draw() const = 0;
virtual std::string to_string() const = 0;
void print() const {
std::cout << to_string() << '\n';
draw();
}
virtual void debug() const = 0;
};
inline Shape::~Shape() { }
inline void Shape::debug() const {
std::cout << "-- デバッグ情報 --\n";
std::cout << "型:" << typeid(*this).name()
<< '\n';
std::cout << "アドレス:" << this << '\n';
}
// 挿入子の多重定義
inline std::ostream& operator<<(
std::ostream& os,
const Shape& s
) {
return os << s.to_string();
}
// 2次元クラス(抽象クラス)
class TwoDimensional {
public:
virtual ~TwoDimensional() = 0;
virtual double get_area() const = 0;
};
inline TwoDimensional::~TwoDimensional() {}
// 直角二等辺三角形クラス(抽象クラス)
class RectEquilTriangle
: public Shape, public TwoDimensional {
protected:
int length;
public:
RectEquilTriangle(int len) : length(len) { }
double get_area() const {
return length * length / 2.0;
}
};
// 左下直角二等辺三角形クラス
class RectEquilTriangleLB
: public RectEquilTriangle {
public:
RectEquilTriangleLB(int len)
: RectEquilTriangle(len) { }
RectEquilTriangleLB* clone() const {
return new RectEquilTriangleLB(length);
}
void draw() const {
for (int i = 1; i <= length; i++) {
for (int j = 1; j <= i; j++)
std::cout << '*';
std::cout << '\n';
}
}
std::string to_string() const {
std::ostringstream os;
os << "RectEquilTriangleLB(length:"
<< length << ")";
return os.str();
}
void debug() const {
Shape::debug();
std::cout << "クラス:RectEquilTriangleLB\n";
std::cout << "アドレス" << this << '\n';
std::cout << "length:" << length << '\n';
}
};
// 右上直角二等辺三角形クラス
class RectEquilTriangleRU
: public RectEquilTriangle {
public:
RectEquilTriangleRU(int len)
: RectEquilTriangle(len) { }
RectEquilTriangleRU* clone() const {
return new RectEquilTriangleRU(length);
}
void draw() const {
for (int i = 1; i <= length; i++) {
for (int j = 1; j <= i - 1; j++)
std::cout << ' ';
for (int j = 1; j <= length - i + 1; j++)
std::cout << '*';
std::cout << '\n';
}
}
std::string to_string() const {
std::ostringstream os;
os << "RectEquilTriangleRU(length:"
<< length << ")";
return os.str();
}
void debug() const {
Shape::debug();
std::cout << "クラス:RectEquilTriangleRU\n";
std::cout << "アドレス" << this << '\n';
std::cout << "length:" << length << '\n';
}
};
int main()
{
Shape* s[] = {
new RectEquilTriangleLB(5), // 左下直角
new RectEquilTriangleRU(4), // 右上直角
};
for (int i = 0; i < sizeof(s) / sizeof(s[0]); i++) {
std::cout << "s[" << i << "]\n";
s[i]->print();
std::cout << '\n';
}
for (int i = 0; i < sizeof(s) / sizeof(s[0]); i++)
delete s[i];
}
// ------------------------------------
以下が、解答のプログラムです。
#include <string>
#include <sstream>
#include <iostream>
// 図形クラス(抽象クラス)
class Shape {
public:
virtual ~Shape() = 0;
virtual Shape* clone() const = 0;
virtual void draw() const = 0;
virtual std::string to_string() const = 0;
void print() const {
std::cout << to_string() << '\n';
draw();
}
virtual void debug() const = 0;
};
inline Shape::~Shape() { }
inline void Shape::debug() const {
std::cout << "-- デバッグ情報 --\n";
std::cout << "型:" << typeid(*this).name()
<< '\n';
std::cout << "アドレス:" << this << '\n';
}
// 挿入子の多重定義
inline std::ostream& operator<<(
std::ostream& os,
const Shape& s
) {
return os << s.to_string();
}
// 2次元クラス(抽象クラス)
class TwoDimensional {
public:
virtual ~TwoDimensional() = 0;
virtual double get_area() const = 0;
};
inline TwoDimensional::~TwoDimensional() {}
// 直角二等辺三角形クラス(抽象クラス)
class RectEquilTriangle
: public Shape, public TwoDimensional {
protected:
int length;
public:
RectEquilTriangle(int len) : length(len) { }
double get_area() const {
return length * length / 2.0;
}
};
// 左下直角二等辺三角形クラス
class RectEquilTriangleLB
: public RectEquilTriangle {
public:
RectEquilTriangleLB(int len)
: RectEquilTriangle(len) { }
RectEquilTriangleLB* clone() const {
return new RectEquilTriangleLB(length);
}
void draw() const {
for (int i = 1; i <= length; i++) {
for (int j = 1; j <= i; j++)
std::cout << '*';
std::cout << '\n';
}
}
std::string to_string() const {
std::ostringstream os;
os << "RectEquilTriangleLB(length:"
<< length << ")";
return os.str();
}
void debug() const {
Shape::debug();
std::cout << "クラス:RectEquilTriangleLB\n";
std::cout << "アドレス" << this << '\n';
std::cout << "length:" << length << '\n';
}
};
// 右上直角二等辺三角形クラス
class RectEquilTriangleRU
: public RectEquilTriangle {
public:
RectEquilTriangleRU(int len)
: RectEquilTriangle(len) { }
RectEquilTriangleRU* clone() const {
return new RectEquilTriangleRU(length);
}
void draw() const {
for (int i = 1; i <= length; i++) {
for (int j = 1; j <= i - 1; j++)
std::cout << ' ';
for (int j = 1; j <= length - i + 1; j++)
std::cout << '*';
std::cout << '\n';
}
}
std::string to_string() const {
std::ostringstream os;
os << "RectEquilTriangleRU(length:"
<< length << ")";
return os.str();
}
void debug() const {
Shape::debug();
std::cout << "クラス:RectEquilTriangleRU\n";
std::cout << "アドレス" << this << '\n';
std::cout << "length:" << length << '\n';
}
};
//
左上直角二等辺三角形クラス
class RectEquilTriangleLU
: public RectEquilTriangle {
public:
RectEquilTriangleLU(int len)
: RectEquilTriangle(len) { }
RectEquilTriangleLU* clone() const {
return new RectEquilTriangleLU(length);
}
void draw() const {
for (int i = length; i >= 1; i--) {
for (int j = 1; j <= i; j++)
std::cout << '*';
std::cout << '\n';
}
std::cout << '\n';
}
std::string to_string() const {
std::ostringstream os;
os << "RectEquilTriangleLU(length:"
<< length << ")";
return os.str();
}
void debug() const {
Shape::debug();
std::cout << "クラス:RectEquilTriangleLU\n";
std::cout << "アドレス" << this << '\n';
std::cout << "length:" << length << '\n';
}
};
//
右下直角二等辺三角形クラス
class RectEquilTriangleRB
: public RectEquilTriangle {
public:
RectEquilTriangleRB(int len)
: RectEquilTriangle(len) { }
RectEquilTriangleRB* clone() const {
return new RectEquilTriangleRB(length);
}
void draw() const {
for (int i = 1; i <= length; i++) {
for (int j = 1; j <= length - i; j++)
std::cout << ' ';
for (int j = 1; j <= i; j++)
std::cout << '*';
std::cout << '\n';
}
std::cout << '\n';
}
std::string to_string() const {
std::ostringstream os;
os << "RectEquilTriangleRB(length:"
<< length << ")";
return os.str();
}
void debug() const {
Shape::debug();
std::cout << "クラス:RectEquilTriangleRB\n";
std::cout << "アドレス" << this << '\n';
std::cout << "length:" << length << '\n';
}
};
int main()
{
Shape* s[] = {
new RectEquilTriangleLB(5), // 左下直角
new RectEquilTriangleRU(4), // 右上直角
new RectEquilTriangleLU(6), //
左上直角
new RectEquilTriangleRB(6), //
右下直角
};
for (int i = 0; i < sizeof(s) / sizeof(s[0]); i++) {
std::cout << "s[" << i << "]\n";
s[i]->print();
std::cout << '\n';
}
for (int i = 0; i < sizeof(s) / sizeof(s[0]); i++)
delete s[i];
}
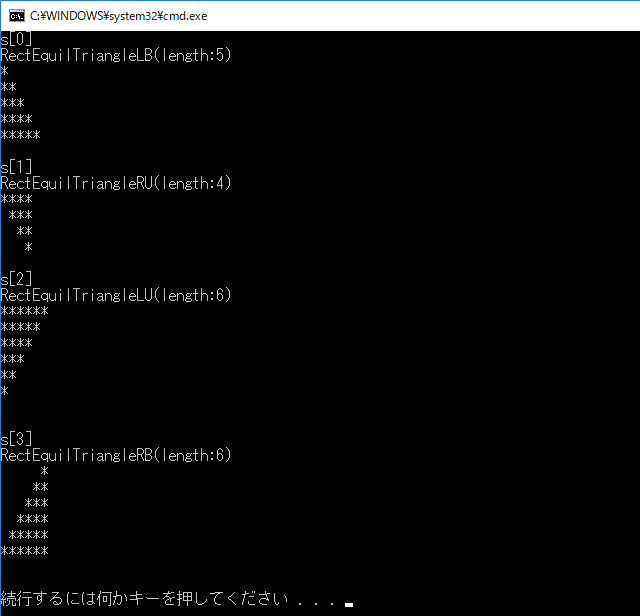
2017年12月30日
《その213》 クロスキャスト(1)
クロスキャスト
下記のプログラムの説明を通して、 クロスキャストの働きを確認したいと思います。
抽象クラス Singer は、
データメンバ name、
純粋仮想デストラクタ ~Singer、
それと、
純粋仮想関数 sing, 関数perform を持っています。
sing は歌う関数です。
(実際には "歌声 ♪" のようなテキストが表示されるだけですが (;^ω^A )
perform は、挨拶してから歌う関数です。
抽象クラス Player は、
純粋仮想デストラクタ ~Player と
関数 play
だけで構成されています。
play は演奏する関数です。
(実際には "演奏 ♪" のようなテキストが表示されるだけですが (;^ω^A )
play の演奏音は、"演奏 ♪ ♪ ♪" です。
Singer_typeAクラス は、クラス Singer の public派生です。
コンストラクタでデータメンバ name を設定します。そして、
関数 sing の歌声を定義します。
sing の歌声は、 "歌声 ♪♪♪♪♪" です。
Singer_typeBクラス は、多重継承クラスです。
クラス Singer,クラス Player の2つから public派生しています。
コンストラクタでデータメンバ name を設定します。そして、
関数 sing の歌声を定義します。
sing の歌声は "歌声 ♪ ♪ ♪" で、Singer_typeA の sing とは少し違っています。
main関数 では、
Singer_typeA の asano と tohno、
Singer_typeB の michi と nakai の4人を定義しています。
そして、Singer*型のポインタ a[i] で4人を指し、
a[i]->perform();
として、動的に 関数 perform を呼び出しています。その結果、
Singer_typeA の2人の歌は、"歌声 ♪♪♪♪♪"
Singer_typeB の2人の歌は、"歌声 ♪ ♪ ♪" となっています。
クラス Player の資産を継承している Singer_typeB の2人は、歌だけでなく演奏もできるのですが、Singer*型のポインタで指されているので、クラス Singer の資産しか使えず、演奏できません。
そこで、 クロスキャスト
dynamic_cast<Player*>(a[i])
を試しています。 Singer*型から Player*型へのキャストです。
キャストに失敗すれば、空ポインタが返されます ★ の箇所の if文は実行されません。
dynamic_cast演算子を用いると、多重継承を行った2つの親へのポインタ間のクロスキャストが成功します。
クロスキャストが成功し、得られた Player*型のポインタ p で
p->play();
とすることで、演奏させることが可能になります。
// ------------------------------------
#include <string>
#include <iostream>
class Singer {
protected:
std::string name;
public:
Singer(std::string str) : name(str) { }
virtual ~Singer() = 0;
virtual void sing() const = 0;
void perform() const {
std::cout << name << "です! よろしく。\n";
sing();
}
};
Singer::~Singer() { }
class Player {
public:
virtual ~Player() = 0;
void play() {
std::cout << "演奏 ♪ ♪ ♪\n";
}
};
Player::~Player() { }
class Singer_typeA : public Singer {
public:
Singer_typeA(std::string str) : Singer(str) { }
void sing() const {
std::cout << "歌声 ♪♪♪♪♪\n";
}
};
class Singer_typeB : public Singer, public Player {
public:
Singer_typeB(std::string str) : Singer(str) { }
void sing() const {
std::cout << "歌声 ♪ ♪ ♪\n";
}
};
int main() {
Singer* a[] = {
new Singer_typeA("asano"),
new Singer_typeA("tohno"),
new Singer_typeB("michi"),
new Singer_typeB("nakai"),
};
for (int i = 0; i < 4; i++) {
a[i]->perform();
/* ★ */ if (Player* p = dynamic_cast<Player*>(a[i]))
p->play();
std::cout << '\n';
delete a[i];
}
}
// ------------------------------------
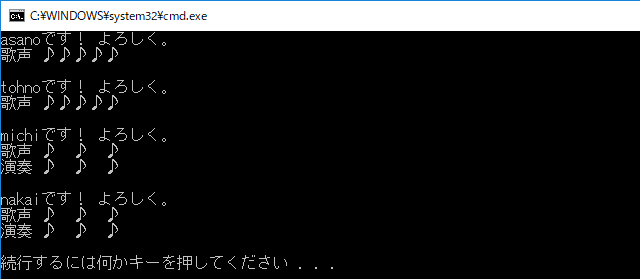
2017年12月29日
《その212》 アップキャストとポインタ & p.245演習7-2
アップキャストとポインタ
下のプログラムにおいて、、
・派生クラス C は、基底クラス A からの 単一継承クラス、
・派生クラス D は、基底クラス A, B からの 多重継承クラスです。
C c; // C型のオブジェクト c を生成
A* ptr1 = &c; // (1)
C* ptr2 = &c; // (2)
(1) 派生オブジェクト c を指すポインタの値 &c を、A* ptr1 に代入しているので、C*型から A*型へのアップキャストが行われています。
(2) &c を C* ptr2 に代入しています。キャストの必要はないので、ptr2 と &c は、当然、同じ値になります。
プログラムの出力結果を見ると、ptr1, ptr2, &c の値は、すべて同じになっています。
D d; // D型のオブジェクト d を生成
A* ptr3 = &d; // (3)
B* ptr4 = &d; // (4)
D* ptr5 = &d; // (5)
(3) 多重継承による派生オブジェクト d を指すポインタの値 &d を、A* ptr3に代入しているので、D*型から A*型へのアップキャストが行われています。
(4) 多重継承による派生オブジェクト d を指すポインタの値 &d を、B* ptr4に代入しているので、D*型から B*型へのアップキャストが行われています。
(5) &d を D* ptr5 に代入しています。キャストの必要はないので、ptr5 と &d は、当然、同じ値になります。
プログラムの出力結果を見ると、多重継承の場合は、ポインタの値が、&dとは違う値に変化する場合があることがわかります。
// ------------------------------------
#include <iostream>
using namespace std;
class A { };
class B { };
class C : public A { };
class D : public A, public B { };
int main() {
C c;
D d;
A* ptr1 = &c; // (1)
C* ptr2 = &c; // (2)
A* ptr3 = &d; // (3)
B* ptr4 = &d; // (4)
D* ptr5 = &d; // (5)
cout << "&c " << &c << '\n';
cout << "(1) " << ptr1 << '\n';
cout << "(2) " << ptr2 << "\n\n";
cout << "&d " << &d << '\n';
cout << "(3) " << ptr3 << '\n';
cout << "(4) " << ptr4 << '\n';
cout << "(5) " << ptr5 << '\n';
}
// ------------------------------------
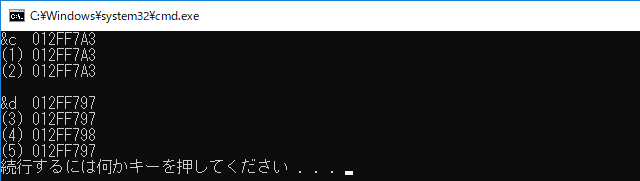
新版明解C++中級編 p.245 演習7-2
次のクラス Derived にメンバ関数 print を追加せよ。なお、メンバ関数 print は、以下の表示を行うものとする。
[1] Base1クラスです : x = 1
[2] Base2クラスです : x = 2
[3] Derivedクラスです : y = 3
なお、[1] と [2] は、下記のプログラムで既に出力されるようになっているので、[3] の表示のみ追加すること。
// ------------------------------------
#include <iostream>
using namespace std;
// 基底クラス1
class Base1 {
public:
int x;
Base1(int a = 0) : x(a) {
cout << "Base1::xを" << x
<< "に初期化しました。\n";
}
void print() {
cout << "Base1クラスです:x = "
<< x << '\n';
}
};
// 基底クラス2
class Base2 {
public:
int x;
Base2(int a = 0) : x(a) {
cout << "Base2::xを" << x
<< "に初期化しました。\n";
}
void print() {
cout << "Base2クラスです:x = "
<< x << '\n';
}
};
// 派生クラス
class Derived
: public Base1, public Base2 {
int y;
public:
Derived(int a, int b, int c)
: y(c), Base2(a), Base1(b) {
cout << "Derived::yを" << y
<< "に初期化しました。\n";
}
void func(int a, int b) {
Base1::x = a;
Base2::x = b;
}
};
int main()
{
Derived z(1, 2, 3);
z.func(1, 2);
z.Base1::print();
z.Base2::print();
}
// ------------------------------------
以下が、解答のプログラムです。
// ------------------------------------
#include <iostream>
using namespace std;
// 基底クラス1
class Base1 {
public:
int x;
Base1(int a = 0) : x(a) {
cout << "Base1::xを" << x
<< "に初期化しました。\n";
}
void print() {
cout << "Base1クラスです:x = "
<< x << '\n';
}
};
// 基底クラス2
class Base2 {
public:
int x;
Base2(int a = 0) : x(a) {
cout << "Base2::xを" << x
<< "に初期化しました。\n";
}
void print() {
cout << "Base2クラスです:x = "
<< x << '\n';
}
};
// 派生クラス
class Derived
: public Base1, public Base2 {
int y;
public:
Derived(int a, int b, int c)
: y(c), Base2(a), Base1(b) {
cout << "Derived::yを" << y
<< "に初期化しました。\n";
}
void func(int a, int b) {
Base1::x = a;
Base2::x = b;
}
void print() {
cout << "Derivedクラスです:y = "
<< y << '\n';
}
};
int main()
{
Derived z(1, 2, 3);
z.func(1, 2);
z.Base1::print();
z.Base2::print();
z.print();
}
// ------------------------------------
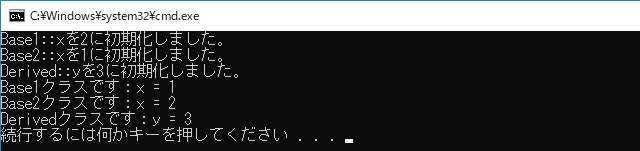
《その211》 多重継承 & p.244演習7-1
多重継承
次の3つのクラスがあるものとします。C は、2つの基底クラス A, B からの派生クラスです。
その結果、C は、2つの基底クラスの資産を継承することになります。このような継承の形を、多重継承と呼びます。
【クラス A】
コンストラクタ … 「 A 」 を表示
デストラクタ … 「~A 」 を表示
データメンバ x = 1
メンバ関数 get_x() … x の値を返却
【クラス B】
コンストラクタ … 「 B 」 を表示
デストラクタ … 「~B 」 を表示
データメンバ x = 1
メンバ関数 get_x() … x の値を返却
【クラス C】 基底クラス A, B を多重継承
コンストラクタ … 「 C 」 を表示
デストラクタ … 「~C 」 を表示
データメンバ x = 1
メンバ関数 get_x() … x の値を返却
次のプログラムの結果から、 コンストラクタが呼び出される順序 と、 デストラクタが呼び出される順序 が確認できます。
また、クラス C は、
基底クラス部分オブジェクト A のデータメンバ x
基底クラス部分オブジェクト B のデータメンバ x
派生クラス C のデータメンバ x
の3つの x を保持することになります。
これらに、get_x関数でアクセスする際には、
c.A::get_x();
c.B::get_x();
c.get_x();
のように、 明確な指定が必要 になります。
// ---------------------------
#include <iostream>
using namespace std;
class A {
protected:
int x;
public:
A() { cout << "A" << '\n'; x = 1; }
~A() { cout << "~A" << '\n'; }
int get_x() const { return x; }
};
class B {
protected:
int x;
public:
B() { cout << "B" << '\n'; x = 2; }
~B() { cout << "~B" << '\n'; }
int get_x() const { return x; }
};
class C : public A, public B {
protected:
int x;
public:
C() { cout << "C" << '\n'; x = 3; }
~C() { cout << "~C" << '\n'; }
int get_x() const { return x; }
};
int main() {
A a;
cout << "------\n";
B b;
cout << "------\n";
C c;
cout << "------\n";
cout << c.A::get_x() << ' '
<< c.B::get_x() << ' '
<< c.get_x() << '\n';
cout << "------\n";
}
// ------------------------------------
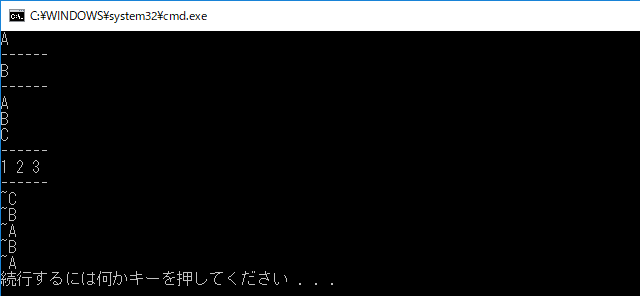
新版明解C++中級編 p.244 演習7-1
以下のクラス群のクラス階層図を描き、クラス Z型のオブジェクトを初期化する際の基底クラス部分オブジェクトの初期化の順序を示せ。
class A{ /* ・・・・・・ */ };
class B{ /* ・・・・・・ */ };
class X : A, B{ /* ・・・・・・ */ };
class Y : B, A{ /* ・・・・・・ */ };
class Z : X, Y{ /* ・・・・・・ */ };
※アクセス指定子 public, protected, private が記述されていない場合は、private派生と解釈されます。
クラス階層図
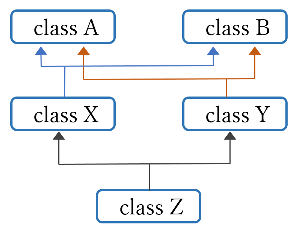
Z型のオブジェクトを初期化する際の規定クラス部分オブジェクトの初期化の順序は、次のプログラムの出力結果の2本の "
"線の間で確認できます。
// ---------------------------
#include <iostream>
class A {
public:
A() { std::cout << "A" << '\n'; }
};
class B {
public:
B() { std::cout << "B" << '\n'; }
};
class X : A, B {
public:
X() { std::cout << "X" << '\n'; }
};
class Y : B, A {
public:
Y() { std::cout << "Y" << '\n'; }
};
class Z : X, Y {
public:
Z() { std::cout << "Z" << '\n'; }
};
int main() {
A a;
B b;
X x;
Y y;
std::cout << "\nZ型のオブジェクトを初期化"
"する際の \n規定クラス部分オ"
"ブジェクトの初期化の順序 \n"
"↓\n------\n";
Z z;
std::cout << "------\n";
}
// ------------------------------------
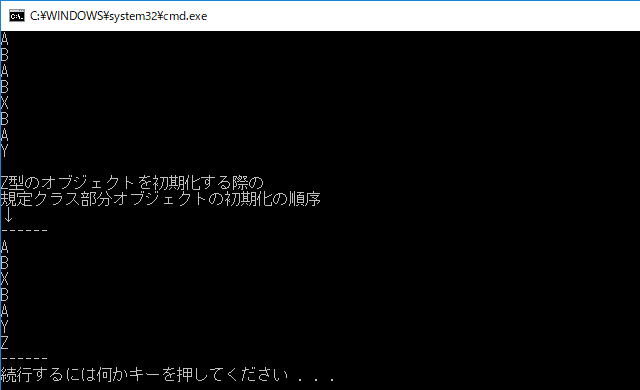
2017年12月28日
《その210》 問題演習 p.235演習6-3
今回も、演習問題です。
新版明解C++中級編 p.235 演習6-3
ジャンケンの《プレーヤ》を表す抽象クラスを定義せよ。そのクラスから、以下のクラスを派生すること。
・人間プレーヤクラス(出す手をキーボードから読み込む)
・コンピュータプレーヤクラス(出す手を乱数で生成する)
以下の解答では、クラス名が次のようになっています。
・JankenPlayer → ジャンケンの《プレーヤ》を表す抽象クラス
・JankenPlayer_A → 人間プレーヤクラス
・JankenPlayer_B → コンピュータプレーヤクラス
// Janken.h
#ifndef ___Janken___
#define ___Janken___
#include<string>
#include<ctime>
#include <cstdlib>
#include <iostream>
int opponent();
class JankenPlayer {
protected:
virtual ~JankenPlayer() { };
int win; // 勝ち回数
int lose; // 負け回数
int draw; // 引分け回数
int te0; // 自分の手
int te1; // 相手の手
public:
// 出す手 te0 を決定 … グー(0), チョキ(1), パー(2)
virtual void set_te0() = 0;
// 判定結果を返却 … 勝ち(0), 負け(1), あいこ(2)
virtual int judge() {
switch (te0) {
case 0 : if (te1 == 0) return 2;
if (te1 == 1) return 0;
if (te1 == 2) return 1;
break;
case 1 : if (te1 == 0) return 1;
if (te1 == 1) return 2;
if (te1 == 2) return 0;
break;
case 2 : if (te1 == 0) return 0;
if (te1 == 1) return 1;
if (te1 == 2) return 2;
break;
}
}
// じゃんけん結果の累積記録
virtual void accumulation(int x){
if (x == 0) win++; // 勝ち → win++
if (x == 1) lose++; // 負け → lose++
if (x == 2) draw++; // あいこ→ draw++
}
// じゃんけんの一連動作
virtual void go() {
// 自分の手を決定
set_te0();
// 相手の手を受け取る
te1 = opponent();
// 勝ち負けの判定
int val = judge();
// 勝負結果の表示
accumulation(val);
if (val == 0) std::cout << "〇勝ち ";
if (val == 1) std::cout << "×負け ";
if (val == 2) std::cout << "△あいこ";
// "グー", "チョキ", "パー" の文字列作成
std::string s0, s1;
switch (te0) {
case 0: s0 = "グー "; break;
case 1: s0 = "チョキ"; break;
case 2: s0 = "パー "; break;
}
switch (te1) {
case 0: s1 = "グー "; break;
case 1: s1 = "チョキ"; break;
case 2: s1 = "パー "; break;
}
// 自分と相手の手を表示
std::cout << "(自分:" << s0 << ", 相手:" << s1 << ")\n";
// 累積結果の表示
std::cout << " 勝ち回数 …" << win << '\n';
std::cout << " 負け回数 …" << lose << '\n';
std::cout << " あいこ回数…" << draw << '\n';
}
};
// 人間プレーヤ
class JankenPlayer_A : public JankenPlayer {
public:
JankenPlayer_A() {
win = 0; lose = 0; draw = 0;
}
// 手の決定 … 勝ち(0), 負け(1), あいこ(2)
virtual void set_te0() {
std::cout << "自分の手を入力"
"《グー(0), チョキ(1), パー(2)》: ";
std::cin >> te0;
}
};
// コンピュータプレーヤ
class JankenPlayer_B : public JankenPlayer {
public:
JankenPlayer_B() {
win = 0; lose = 0; draw = 0;
srand((unsigned)time(NULL));
}
// 手の決定 … 勝ち(0), 負け(1), あいこ(2)
virtual void set_te0() {
te0 = rand() % 3;
}
};
// 対戦相手
int opponent() {
return rand() % 3;
}
#endif
// ------------------------------------
// Janken.cpp
#include <iostream>
#include "Janken.h"
using namespace std;
int main() {
int x;
cout << "あなたは、人間プレーヤ(0)ですか?\n"
" コンピュータプレーヤ(1)ですか?"
" (\"0\" or \"1\" を入力) : ";
cin >> x;
JankenPlayer_A playerA;
JankenPlayer_B playerB;
char q;
do {
cout << "\n◆開始!\n";
if (!x) playerA.go();
else playerB.go();
cout << "続けますか(\"Y\" or \"N\") : "; cin >> q;
} while (q != 'N' && q != 'n');
}
// ------------------------------------
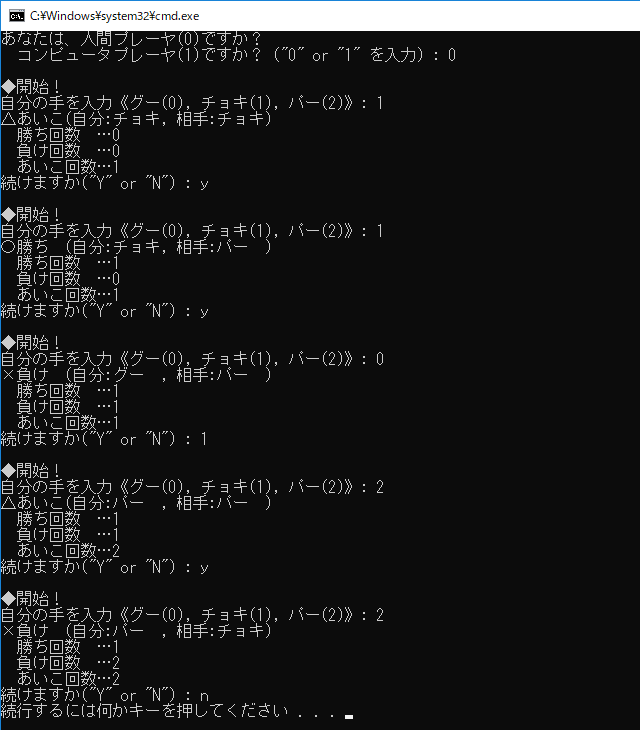
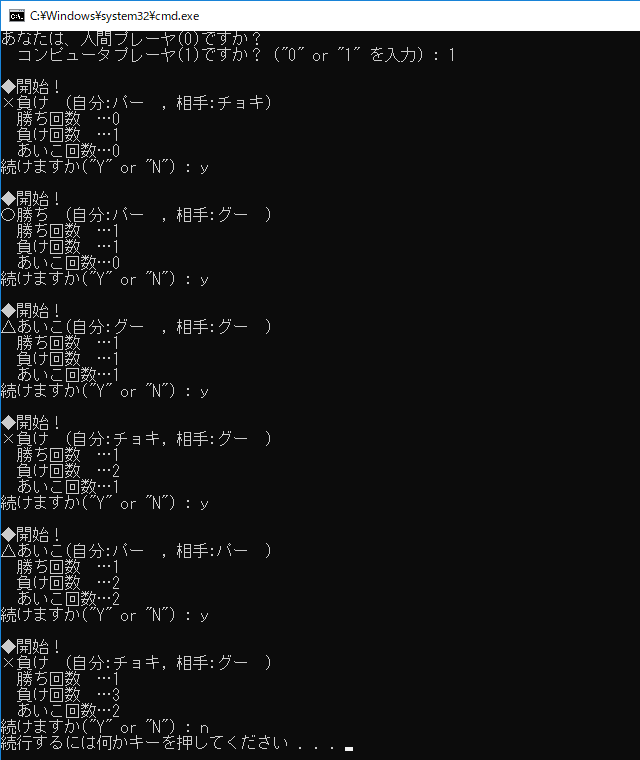
2017年12月27日
《その209》 問題演習 p.235演習6-2
今回も、前回《208》の続きの演習問題です。
新版明解C++中級編 p.235 演習6-2
前回《208》の 演習6-1 で作成したクラスを含め、すべての図形クラス群をテストするプログラムを作成せよ。ただし、各図形の寸法は、キーボードから読み込むようにすること。
// Shape.h
#ifndef ___Class_Shape
#define ___Class_Shape
#include <string>
#include <sstream>
#include <iostream>
// 図形クラス(抽象クラス)
class Shape {
public:
virtual ~Shape() = 0;
// コピー作製
virtual Shape* clone() const = 0;
// 図形出力
virtual void draw() const = 0;
// 出力用文字列作成
virtual std::string to_string() const = 0;
// to_string() の返却値出力 と draw()による図形出力
void print() const {
std::cout << to_string() << '\n';
draw();
}
// デバッグ用情報表示
virtual void debug() const;
};
inline Shape::~Shape() { }
inline void Shape::debug() const
{
std::cout << "-- デバッグ情報 --\n";
std::cout << "型 : " << typeid(*this).name() << '\n';
std::cout << "アドレス : " << this << '\n';
std::cout << "------------------\n";
}
// 点クラス
class Point : public Shape {
public:
void draw() const {
std::cout << "*\n";
}
Point* clone() const {
return new Point;
}
std::string to_string() const {
return "Point";
}
void debug() const {
Shape::debug();
}
};
// 直線クラス(抽象クラス)
class Line : public Shape {
protected:
int length;
public:
Line(int len) : length(len) { }
int get_length() const { return length; }
void set_length(int len) { length = len; }
};
// 横直線クラス
class HorzLine : public Line {
public:
HorzLine(int len) : Line(len) { }
HorzLine* clone() const {
return new HorzLine(length);
}
void draw() const {
for (int i = 1; i <= length; i++)
std::cout << '-';
std::cout << '\n';
}
std::string to_string() const {
std::ostringstream os;
os << "HorzLine(length:" << length << ")";
return os.str();
}
};
// 縦直線クラス
class VertLine : public Line {
public:
VertLine(int len) : Line(len) { }
VertLine* clone() const {
return new VertLine(length);
}
void draw() const {
for (int i = 1; i <= length; i++)
std::cout << "|\n";
}
std::string to_string() const {
std::ostringstream os;
os << "VertLine(length:" << length << ")";
return os.str();
}
};
// 長方形クラス
class Rectangle : public Shape {
int width;
int height;
public:
Rectangle(int w, int h)
: width(w), height(h) { }
Rectangle* clone() const {
return new Rectangle(width, height);
}
void draw() const {
for (int i = 1; i <= height; i++) {
for (int j = 1; j <= width; j++)
std::cout << '*';
std::cout << '\n';
}
}
std::string to_string() const {
std::ostringstream os;
os << "Rectangle(width:" << width
<< ", height:" << height << ")";
return os.str();
}
};
// 直角二等辺三角形クラス(抽象クラス)
class R_angl_triangle
: public Shape {
protected:
int length;
public:
R_angl_triangle(int len) : length(len) { }
int get_length() const { return length; }
void set_length(int len) { length = len; }
};
// 左下直角の三角形
class R_angl_l_under :
public R_angl_triangle {
public:
R_angl_l_under(int len)
: R_angl_triangle(len) { }
R_angl_l_under* clone() const {
return new R_angl_l_under(length);
}
void draw() const {
for (int i = 1; i <= length; i++) {
for (int j = 1; j <= i; j++)
std::cout << '*';
std::cout << '\n';
}
}
std::string to_string() const {
std::ostringstream os;
os << "R_angl_l_under(length:"
<< length << ")";
return os.str();
}
};
// 左上直角の三角形
class R_angl_l_upper
: public R_angl_triangle {
public:
R_angl_l_upper(int len)
: R_angl_triangle(len) { }
R_angl_l_under* clone() const {
return new R_angl_l_under(length);
}
void draw() const {
for (int i = length; i >= 1; i--) {
for (int j = 1; j <= i; j++)
std::cout << '*';
std::cout << '\n';
}
std::cout << '\n';
}
std::string to_string() const {
std::ostringstream os;
os << "R_angl_l_upper(length:"
<< length << ")";
return os.str();
}
};
// 右下直角の三角形
class R_angl_r_under
: public R_angl_triangle {
public:
R_angl_r_under(int len)
: R_angl_triangle(len) { }
R_angl_r_under* clone() const {
return new R_angl_r_under(length);
}
void draw() const {
for (int i = 1; i <= length; i++) {
for (int j = 1; j <= length - i; j++)
std::cout << ' ';
for (int j = 1; j <= i; j++)
std::cout << '*';
std::cout << '\n';
}
std::cout << '\n';
}
std::string to_string() const {
std::ostringstream os;
os << "R_angl_r_under(length:"
<< length << ")";
return os.str();
}
};
// 右上直角の三角形
class R_angl_r_upper
: public R_angl_triangle {
public:
R_angl_r_upper(int len)
: R_angl_triangle(len) { }
R_angl_r_upper* clone() const {
return new R_angl_r_upper(length);
}
void draw() const {
for (int i = 1; i <= length; i++) {
for (int j = 1; j <= i - 1; j++)
std::cout << ' ';
for (int j = 1; j <= length - i + 1; j++)
std::cout << '*';
std::cout << '\n';
}
}
std::string to_string() const {
std::ostringstream os;
os << "R_angl_r_upper(length:"
<< length << ")";
return os.str();
}
};
// 挿入子「 << 」の多重定義
inline std::ostream& operator<<(
std::ostream& os,
const Shape& s
)
{
return os << s.to_string();
}
#endif
// ------------------------------------
// ShapeTest.cpp
#include <iostream>
#include "Shape.h"
using namespace std;
int main() {
int x1, x2, x3, x4, x5;
cout << "次の寸法を入力してください。\n"
" ・横直線の長さ\n"
" ・縦直線の長さ\n"
" ・長方形の横幅・高さ\n"
" ・直角二等辺三角形の直角をはさむ辺の長さ\n\n";
cout << " 入力・横直線の長さ : "; cin >> x1;
cout << " 入力・縦直線の長さ : "; cin >> x2;
cout << " 入力・長方形の横幅 : "; cin >> x3;
cout << " 入力・ 高さ : "; cin >> x4;
cout << " 入力・直角二等辺三角形の直角をはさむ"
"辺の長さ : "; cin >> x5;
Shape* a[] = {
new Point(),
new HorzLine(x1),
new VertLine(x2),
new Rectangle(x3, x4),
new R_angl_l_under(x5),
new R_angl_l_upper(x5),
new R_angl_r_under(x5),
new R_angl_r_upper(x5),
};
for (int i = 0; i < sizeof(a) / sizeof(a[0]); i++) {
cout << "\n◆ "<< i + 1 << ".\n";
a[i]->print();
a[i]->debug();
cout << '\n';
}
for (int i = 0; i < sizeof(a) / sizeof(a[0]); i++)
delete a[i];
}
// ------------------------------------
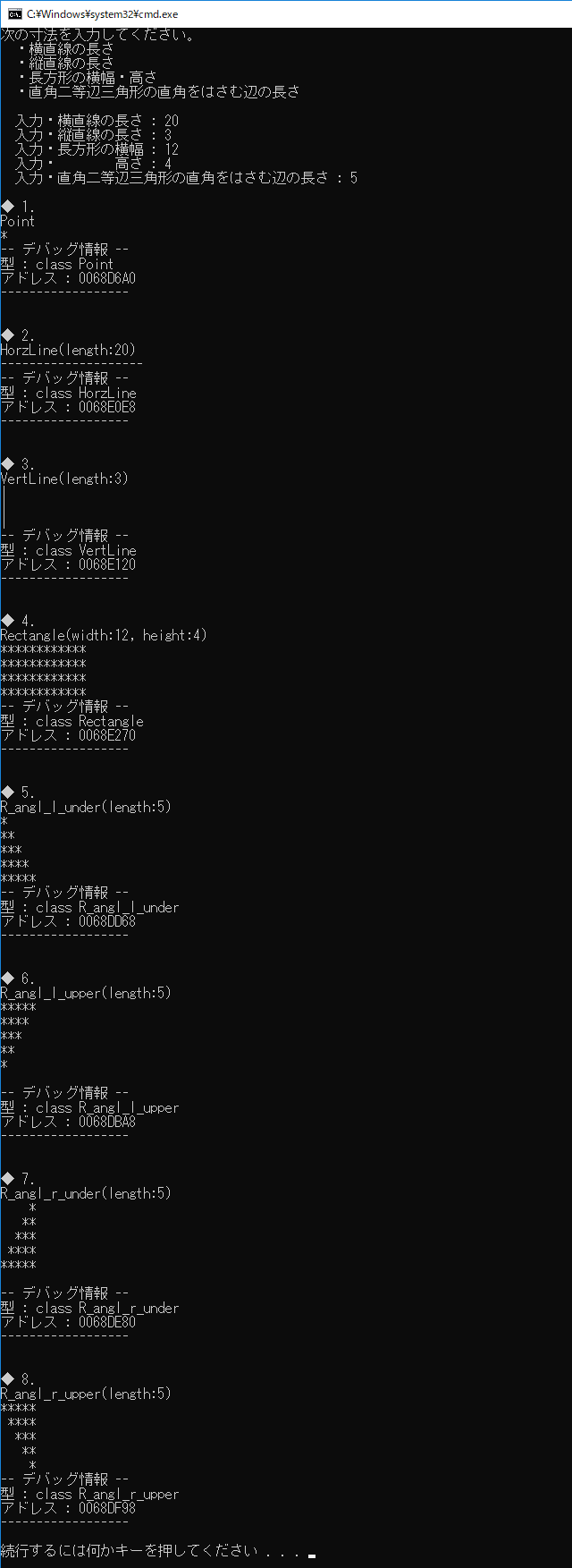
《その208》 問題演習 p.235演習6-1
以下は、今回の演習問題の課題となる図形クラス群です。
・図形クラス(抽象クラス) Shape
から派生した
・点クラス Point
・直線クラス(抽象クラス) Line
・長方形クラス Rectangle
さらに、直線クラス(抽象クラス) Line から派生した
・横線クラス HorzLine
・縦線クラス VertLine
で、構成されています。
// ------------------------------------
#ifndef ___Class_Shape
#define ___Class_Shape
#include <string>
#include <sstream>
#include <iostream>
// 図形クラス(抽象クラス)
class Shape {
public:
virtual ~Shape() = 0;
// コピー作製
virtual Shape* clone() const = 0;
// 図形出力
virtual void draw() const = 0;
// 出力用文字列作成
virtual std::string to_string() const = 0;
// to_string() の返却値出力 と draw()による図形出力
void print() const {
std::cout << to_string() << '\n';
draw();
}
// デバッグ用情報表示
virtual void debug() const = 0;
};
inline Shape::~Shape() { }
inline void Shape::debug() const
{
std::cout << "-- デバッグ情報 --\n";
std::cout << "型 : " << typeid(*this).name() << '\n';
std::cout << "アドレス : " << this << '\n';
}
// 点クラス
class Point : public Shape {
public:
void draw() const {
std::cout << "*\n";
}
Point* clone() const {
return new Point;
}
std::string to_string() const {
return "Point";
}
void debug() const {
Shape::debug();
}
};
// 直線クラス(抽象クラス)
class Line : public Shape {
protected:
int length; // 長さ
public:
Line(int len) : length(len) { }
int get_length() const { return length; }
void set_length(int len) { length = len; }
void debug() const {
Shape::debug();
std::cout << "長さ : " << length << '\n';
}
};
// 横直線クラス
class HorzLine : public Line {
public:
HorzLine(int len) : Line(len) { }
virtual HorzLine* clone() const {
return new HorzLine(length);
}
void draw() const {
for (int i = 1; i <= length; i++)
std::cout << '-';
std::cout << '\n';
}
std::string to_string() const {
std::ostringstream os;
os << "HorzLine(length:" << length << ")";
return os.str();
}
};
// 縦直線クラス
class VertLine : public Line {
public:
VertLine(int len) : Line(len) { }
virtual VertLine* clone() const {
return new VertLine(length);
}
void draw() const {
for (int i = 1; i <= length; i++)
std::cout << "|\n";
}
std::string to_string() const {
std::ostringstream os;
os << "VertLine(length:" << length << ")";
return os.str();
}
};
// 長方形クラス
class Rectangle : public Shape {
int width; // 横幅
int height; // 高さ
public:
Rectangle(int w, int h)
: width(w), height(h) { }
Rectangle* clone() const {
return new Rectangle(width, height);
}
void draw() const {
for (int i = 1; i <= height; i++) {
for (int j = 1; j <= width; j++)
std::cout << '*';
std::cout << '\n';
}
}
std::string to_string() const {
std::ostringstream os;
os << "Rectangle(width:" << width
<< ", height:" << height << ")";
return os.str();
}
void debug() const {
Shape::debug();
std::cout << "横幅 : " << width << '\n';
std::cout << "高さ : " << height << '\n';
}
};
// 挿入子「 << 」の多重定義
inline std::ostream& operator<<(
std::ostream& os,
const Shape& s
)
{
return os << s.to_string();
}
#endif
// ------------------------------------
新版明解C++中級編 p.235 演習6-1
上記の図形クラス群に対して、直角二等辺三角形を表すクラス群を追加せよ。左下が直角のもの、左上が直角のもの、右下が直角のもの、右上が直角のものを追加すること。直角二等辺三角形を表す抽象クラスを作り、そこから個々のクラスを派生して作ること。
以下が、解答のクラス群です。
・直角二等辺三角形クラス(抽象クラス) R_angl_triangle
と、この抽象クラスから派生した
・左下が直角の直角二等辺三角形クラス R_angl_l_under
・左上が直角の直角二等辺三角形クラス R_angl_l_upper
・右下が直角の直角二等辺三角形クラス R_angl_r_under
・右上が直角の直角二等辺三角形クラス R_angl_r_upper
の構成です。
// 直角二等辺三角形クラス(抽象クラス)
class R_angl_triangle
: public Shape {
protected:
int length; // 直角をはさむ辺の長さ
public:
R_angl_triangle(int len) : length(len) { }
int get_length() const { return length; }
void set_length(int len) { length = len; }
void debug() const {
Shape::debug();
std::cout << "length : "
<< length << '\n';
}
};
// 左下直角の三角形
class R_angl_l_under :
public R_angl_triangle {
public:
R_angl_l_under(int len)
: R_angl_triangle(len) { }
virtual R_angl_l_under* clone() const {
return new R_angl_l_under(length);
}
void draw() const {
for (int i = 1; i <= length; i++) {
for (int j = 1; j <= i; j++)
std::cout << '*';
std::cout << '\n';
}
}
std::string to_string() const {
std::ostringstream os;
os << "R_angl_l_under(length:"
<< length << ")";
return os.str();
}
};
// 左上直角の三角形
class R_angl_l_upper
: public R_angl_triangle {
public:
R_angl_l_upper(int len)
: R_angl_triangle(len) { }
virtual R_angl_l_under* clone() const {
return new R_angl_l_under(length);
}
void draw() const {
for (int i = length; i >= 1; i--) {
for (int j = 1; j <= i; j++)
std::cout << '*';
std::cout << '\n';
}
std::cout << '\n';
}
std::string to_string() const {
std::ostringstream os;
os << "R_angl_l_upper(length:"
<< length << ")";
return os.str();
}
};
// 右下直角の三角形
class R_angl_r_under
: public R_angl_triangle {
public:
R_angl_r_under(int len)
: R_angl_triangle(len) { }
virtual R_angl_r_under* clone() const {
return new R_angl_r_under(length);
}
void draw() const {
for (int i = 1; i <= length; i++) {
for (int j = 1; j <= length - i; j++)
std::cout << ' ';
for (int j = 1; j <= i; j++)
std::cout << '*';
std::cout << '\n';
}
std::cout << '\n';
}
std::string to_string() const {
std::ostringstream os;
os << "R_angl_r_under(length:"
<< length << ")";
return os.str();
}
};
// 右上直角の三角形
class R_angl_r_upper
: public R_angl_triangle {
public:
R_angl_r_upper(int len)
: R_angl_triangle(len) { }
virtual R_angl_r_upper* clone() const {
return new R_angl_r_upper(length);
}
void draw() const {
for (int i = 1; i <= length; i++) {
for (int j = 1; j <= i - 1; j++)
std::cout << ' ';
for (int j = 1; j <= length - i + 1; j++)
std::cout << '*';
std::cout << '\n';
}
}
std::string to_string() const {
std::ostringstream os;
os << "R_angl_r_upper(length:"
<< length << ")";
return os.str();
}
};
2017年12月26日
《その207》 抽象クラス(5)
抽象クラス
今回のプログラムは、基本的な事項のまとめ的なものです。
図形の形状別に、
長方形クラス Rectangle
円クラス Circle
三角形クラス Triangle
があって、それぞれの基底クラスは 抽象クラス Area です。
プログラムの簡単な説明を、コード中にコメントとして入れました。
// ------------------------------------
#include <sstream>
#include <string>
#include <iostream>
// 面積クラス(抽象クラス) Area
class Area {
public:
// 仮想デストラクタ
virtual ~Area() { }
// 純粋仮想関数(面積を計算する)
virtual double area() const = 0;
// 純粋仮想関数(形状を返す)
virtual std::string shape() const = 0;
// 純粋仮想関数(出力用の文字列を作る)
virtual std::string to_string() const = 0;
};
// 長方形クラス
class Rectangle : public Area {
double width;
double height;
public:
Rectangle(double x, double y)
: width(x), height(y) { }
double area() const {
return width * height;
}
std::string shape() const {
return "長方形";
}
std::string to_string() const {
std::ostringstream os;
os << shape() << "\n横幅 : "
<< width << " 高さ : "
<< height << '\n'
<< "面積 : " << area()
<< '\n';
return os.str();
}
};
// 円クラス
class Circle : public Area {
double radius;
public:
Circle(double x) : radius(x) { }
double area() const {
return radius * radius * 3.14;
}
std::string shape() const {
return "円";
}
std::string to_string() const {
std::ostringstream os;
os << shape() << "\n半径 : "
<< radius << "\n面積 : "
<< area() << '\n';
return os.str();
}
};
// 三角形クラス
class Triangle : public Area {
double base;
double height;
public:
Triangle (double x, double y)
: base(x), height(y) { }
double area() const {
return base * height / 2;
}
std::string shape() const {
return "三角形";
}
std::string to_string() const {
std::ostringstream os;
os << shape() << "\n底辺 : "
<< base << " 高さ : "
<< height << '\n'
<< "面積 : " << area()
<< '\n';
return os.str();
}
};
// 挿入子「 << 」の多重定義
// ※挿入子多重定義用関数 operator<<
// の第1引数を ostream& にする必要
// があるため、Areaクラスのメンバ関
// 数として定義することができません。
// ※この operator<<関数を、 ヘッダ内に
// 置く場合には、inline を付けて内部
// 結合を与える 必要があります。
std::ostream& operator<<(
std::ostream& os,
const Area& a
)
{
return os << a.to_string();
}
// main関数
int main() {
// Area* を使ってすべての派生クラスを指
// すことが可能 です。
Area* a[] = {
new Rectangle(12.3, 2.0),
new Circle(2.0),
new Circle(5.0),
new Triangle(5, 3),
};
for (int i = 0; i < 4; i++) {
std::cout << *a[i] << '\n';
delete a[i];
}
}
// ------------------------------------
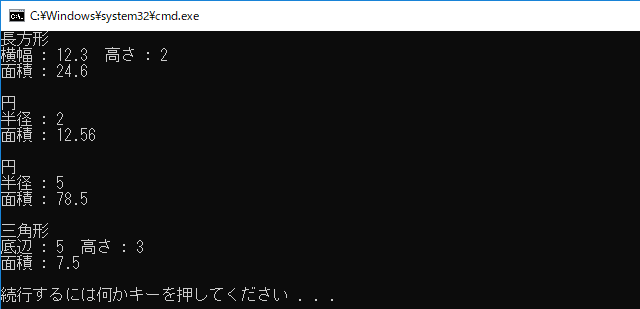
《その206》 抽象クラス(4)
抽象クラス
最近、抽象クラスの話が続いてしまっていて、すみません (ノω<。) m(_ _)m
今回もまた、抽象クラスです。
前回《205》の内容のまとめです ( ̄▽ ̄;)!!
下のプログラムは、前回《205》のプログラムから余計な部分を取り除いた構成になっています。
◆1. 動的にオブジェクト Bbb(5) を作り、そのオブジェクトへのポインタを Aaa*型の q に代入します。
オブジェクト Bbb(5) は Bbb型なので、Bbb*型のポインタで指せるのは当然ですが、派生クラス Bbb の基底クラスが Aaa なので、アップキャストにより、Aaa*型のポインタで指すことができます。
Aaa は仮想関数を持つ多相的クラスなので、Bbb も多相的クラスですから、多相性の利用といった点からも Aaa*型のポインタを利用するのが自然です。
◆2. Bbb型オブジェクトの配列用の記憶域を確保して、その先頭要素へのポインタを int* p に代入します。
◆3. 作成した Bbb型オブジェクトを破棄する支持です。
◆4. この Bbb型オブジェクトを指すポインタ q が Aaa*型なので、デストラクタ Aaa::~Aaa が呼ばれるはずですが、~Aaa は仮想デストラクタですから、Bbbクラスのデストラクタ ~Bbb が呼ばれることになります。その結果、動的に確保した配列領域が解放されます。
◆5. 派生クラスのデストラクタ ~Bbb の次に、基底クラスのデストラクタ ~Aaa が呼ばれます。
◆6. もし、プログラムの ◆5. のコードが、 ◆6. のコードになっていたら、~Aaa が呼ばれ、~Bbb は呼ばれないままになってしまいます。その結果、動的に確保した配列領域が解放されないままになってしまいます。
// ------------------------------------
#include <iostream>
class Aaa {
public:
virtual ~Aaa() { // ◆5.
// ~Aaa() { // ◆6.
std::cout << "~Aaa が呼ばれました。\n";
}
virtual void f1() const = 0;
// 「 = 0 」 を付けて純粋仮想関数にしてあるので、
// 関数本体がどこかで定義されるま
// では、コンパイルが成功しません。
// vittual void f1() const { }
// のような、純粋でない仮想関数であれば
// 問題なくコンパイルは成功します。
// ということは、純粋仮想関数にするこ
// とで、関数本体の定義を、プログラマー
// に促すことができるわけです。
};
class Bbb : public Aaa {
int n;
int* p;
public:
Bbb(int x) : n(x) {
p = new int[n]; // ◆2.
}
~Bbb() { // ◆4.
delete[] p;
std::cout << "~Bbb が呼ばれました。\n";
}
void f1() const{ /* 関数 f1 の本体 */ }
void f2() { /* 関数 f2 の本体 */ }
};
int main() {
Aaa* q = new Bbb(5); // ◆1.
delete q; // ◆3.
}
// ------------------------------------

2017年12月25日
《その205》 抽象クラス(3)
抽象クラス
下記のプログラムでは、抽象クラス Aaa は3つの純粋仮想関数 set, get, func を持っています。
純粋仮想関数は、関数 set, 関数 get のように、関数本体を与えずに記述するのが一般的ですが、
関数 func のように関数本体を定義することも可能です( ★1. と ★2. )。
関数 Aaa::func は、関数 set と 関数 get を連続して呼び出します。
純粋指定子「 = 0 」 ★1. を付ける都合上、関数定義をクラス定義の外に記述してあります ★2. 。
また、抽象クラス Aaa のデストラクタ ~Aaa ★3. は virtual の付いた仮想デストラクタになっています。
virtual無しの場合は、プログラム終了時に、デストラクタ ~Bbb が呼ばれず、 動的に確保 ★4. した領域が解放されないままになってしまいます。
// ------------------------------------
#include <iostream>
class Aaa {
public:
virtual ~Aaa() { // ★3.
std::cout << "~Aaa が呼ばれました。\n";
}
virtual void set() = 0;
virtual void get() const = 0;
virtual void func() = 0; // ★1.
};
void Aaa::func() { // ★2.
set(); get();
}
class Bbb : public Aaa{
int n;
int* p;
public:
Bbb(int x) : n(x) {
p = new int[n]; // ★4.
}
~Bbb() {
delete[] p;
std::cout << "~Bbb が呼ばれました。\n";
}
void set() {
for (int i = 0; i < n; i++)
p[i] = i;
}
void get() const {
for (int i = 0; i < n; i++)
std::cout << p[i] << " ";
std::cout << '\n';
}
void func() { Aaa::func(); }
};
int main() {
Aaa* p = new Bbb(5);
p->func();
delete p;
}
// ------------------------------------

もし、 ★3. の箇所で、デストラクタ ~Aaa に virtual が付いていない場合は、下のような結果になります。
デストラクタ ~Bbb が呼ばれていないことが確認できます。
